Environment Setup and Source Code
As prerequisite we will need to get Eclipse SDK and add the E4 Tools.
- in this tutorial we use Eclipse IDE for Eclipse Committers 4.5.0 M5 plus the E4 Tools I20150105-1100.
- The source code for this examples is in project com.asegno.e4app.first , clonable from https://github.com/psuzzi/asegno.git repo.
Create an E4 RCP Application
CTRL+N > type “4” , select Eclipse 4 Application Project, click Next.
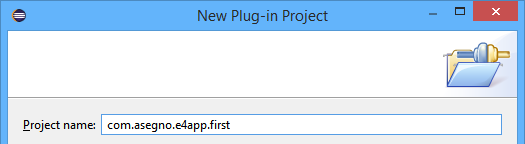
Type com.asegno.e4app.first as Project Name, keep defaults and press Next

Check “Generate an activator ..” , keep other defaults and press Next.

Check “Add a lifecycle class”, keep other defaults and click Finish.
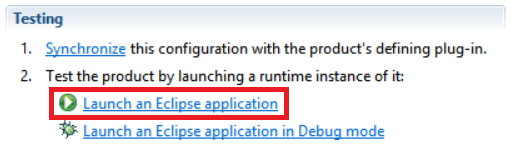
Launch the application from the product file to check it can run.
Edit the @PostContextCreate method in the E4 Lifecycle class
/**
* Note : the model has not been loaded at this point
*/
@PostContextCreate
void postContextCreate(IEclipseContext workbenchContext) {
final Shell shell = new Shell(SWT.TOOL|SWT.NO_TRIM);
LoginDialog dialog = new LoginDialog(shell);
if(dialog.open() != Window.OK){
// close application
System.exit(-1);
}
// continue to the initial window
}
Then add a LoginDialog class as follows
public class LoginDialog extends TitleAreaDialog {
// Widgets
private Text textUsername;
private Text textPassword;
@Inject
MApplication application;
public LoginDialog(Shell parentShell) {
super(parentShell);
}
@Override
protected Control createContents(Composite parent) {
Control contents = super.createContents(parent);
setTitle("Login");
setMessage("Please provide credentials");
return contents;
}
@Override
protected Control createDialogArea(Composite parent) {
Composite area = (Composite) super.createDialogArea(parent);
System.out.println(application);
Composite container = new Composite(area, SWT.NULL);
container.setLayout(new GridLayout(2, false));
container.setLayoutData(new GridData(GridData.FILL_BOTH));
new Label(container, SWT.NULL).setText("Username");
textUsername = new Text(container, SWT.BORDER);
textUsername.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
new Label(container, SWT.NULL).setText("Password");
textPassword = new Text(container, SWT.PASSWORD | SWT.BORDER);
textPassword.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
return area;
}
@Override
protected Point getInitialSize() {
return new Point(500, 300);
}
}
If you launch the application you’ll see the the System.out.println(application) will print null. This is because the LoginDialog is launched @PostContextCreate, when the model is not yet loaded.
But, if you open the dialog in the @ProcessAdditions, and you invoke the ContextInjectionFactory.inject(dialog, workbenchContext);
before opening the dialog, you’ll see the application is not null anymore. Therefore edit the two methods, as below
@PostContextCreate
void postContextCreate(IEclipseContext workbenchContext) {
}
/** ... */
@ProcessAdditions
void processAdditions(IEclipseContext workbenchContext) {
final Shell shell = new Shell(SWT.TOOL|SWT.NO_TRIM);
LoginDialog dialog = new LoginDialog(shell);
ContextInjectionFactory.inject(dialog, workbenchContext);
if(dialog.open() != Window.OK){
// close application
System.exit(-1);
}
// continue to the initial window
}
So, we’re going to add an hook to the APP_STARTUP_COMPLETE for getting isntances of Application’s object and services.
First, add plugins needed to use the event framework
- org.eclipse.e4.core.services
- org.eclipse.osgi.services
References
- Kai Toeder on lifecycle hooks
- Eclipse Bugzilla’s bug 376821
- Eclipse Forum on login dialog and lifecycle
- Vogella on E4 Event System
0 Comments