Here are three examples covering maven basics in Eclipse such as: convert a java project to maven nature, create a project with an archetype selection, and refresh/update projects and understand files and structure.
For the article, I use eclipse-jee-2019-06, which already provides maven support. If you use a different version of eclipse, ensure the m2e plugin is installed (+ m2e-wtp for java-web projects)
Adding dependencies
If you want IDE suggestions for adding maven dependencies from maven central, make sure you download the maven index at least once. Otherwise, you should find yourself the dependencies from maven central or from your company’s nexus.
For downloading the maven index, use Eclipse Preferences > Maven, then check Download Repository index updates at startup and restart eclipse.
Creating a Maven Project
In this section, we see three basic use cases when using maven in Eclipse:
- Convert an existing java project to maven nature
- Create a simple maven project using the default archetype
- Create a maven project with archetype selection (maven-achetype-webapp)
Add maven nature to Java project
If you have a plain java project, you can convert it to maven nature as follows. Select the java project, right-click on it, then select Configure > Convert to Maven Project. Then, you will see a dialog for adding all the relevant information to transform the java project into a maven one.
The dialog provides some reasonable defaults for version and packaging. So, to complete the conversion to maven, you just need to fill the mandatory fields: Group Id and Artifact Id, and then click on Finish.
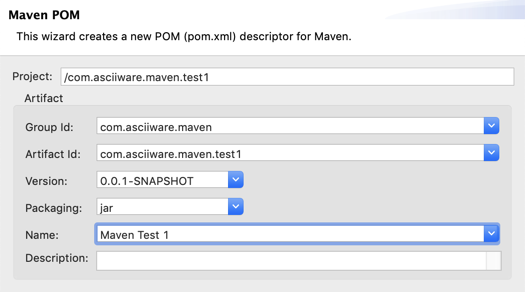
When done, the wizard converted the java project into a java-maven project. The conversion consists of adding a pom.xml, adding the maven nature into a hidden .project file, and change the default output folder according to maven defaults.
After conversion, there are a few changes in the project folder. First, notice the pom.xml
in the project’s root. Then, consider that, from now on, all the .class files are compiled in the maven default output folder: target/classes/
( in opposition to bin/
which is the default for Java projects)
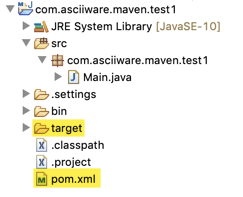
Be aware that the project source folder is unchanged, so it still is src/
: default source folder for java projects, but not for maven. As consequence, your pom.xml explicitly declares the sourceDirectory
as follows.
<sourceDirectory>src</sourceDirectory>
To see the differences before and after the conversion, display hidden files (filter .* resources) and look at how .classpath
and .project
are changed.
Quickstart new java-Maven project
This is the quickest way to build a standard java-maven project in Eclipse.
Open the new.. wizard with Ctrl+N
and type “maven”. Select Maven Project, and proceed with Next.
In the next Dialog page, select Create a simple project (skip archetype selection) and press Next.

In the next page, fill the Group id, and the Artifact Id as you see below and then click Finish.
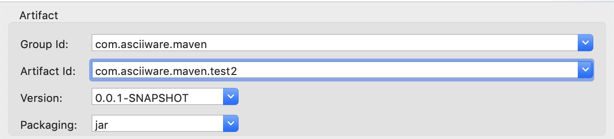
You just generated a “proper” java-maven project with a pom.xml and source folders as per maven defaults.
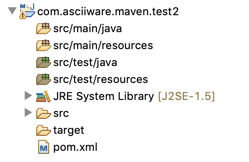
Note: the project is configured with java 1.5, despite my eclipse workspace is configured with a different java version. In order to configure a specific java version, you either need to set the maven.compiler.source
and maven.compiler.target
properties or configure the maven-compiler-plugin
(see also: maven documentation).
Here we go by setting the pom.xml properties as you see below.
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
After the change we need to update the project, so maven and eclipse are in sync. To do so, select the project, right-click and choose Maven > Update Project.
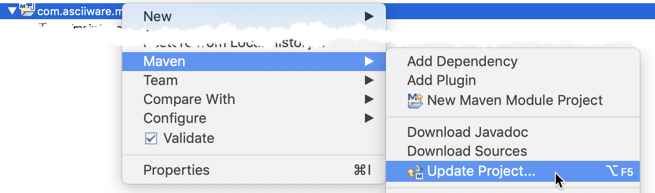
After the update, you can see the project is using the desired JRE version.
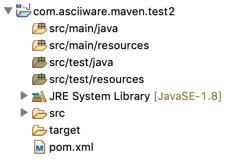
Create a new Java-web maven project
This is an example of creating a java-maven project with archetype selection. In this case, we create a java web project.
Open the new.. wizard with Ctrl+N
and type maven. Select Maven Project, and proceed with Next.
In the next dialog press Next. In the following page, choose the maven archetype for java web app: maven-archetype-webapp
, and click Next.

In the next page, fill the Group id, and the Artifact Id, adjust the Package if needed, and then click Finish.
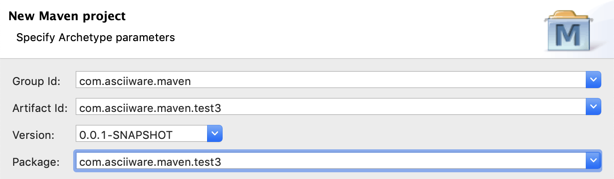
After the project is generated, you see the generated project as visible below.
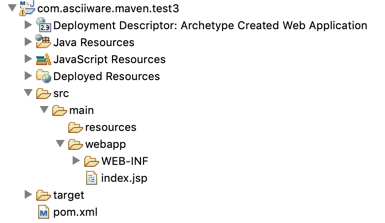
Note: after generating the project, you might see a JSP Problem: The superclass “javax.servlet.http.HttpServlet” was not found on the Java Build Path. To solve this error, just open the project properties, select Targeted Runtimes, and set your servlet container as a target runtime.
0 Comments