Here is a quick introduction on how to create a Spring Boot web app.
For this tutorial, I use Spring Tools 4 for Eclipse.
Spring Boot
Spring is a flexible framework with a lot of components that can be configured to be used by you Java applications.
SpringBoot is a convention over confguration framework providing reasonable defaults to simplify the configuration of a Spring application.
Initialize a Web App
Create a new Spring Starter Project]

Use com.asciiware.spring.web.app as Name, Group, and Package and click Next.
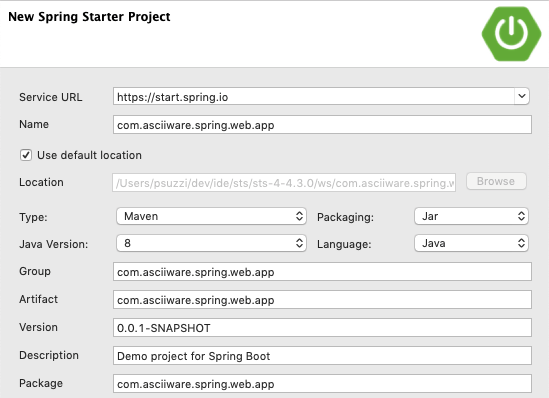
In the next page, add the following dependencies.
- Spring Web Starter: to build web/REST application, also embeds Tomcat
- Thymeleaf: add server-side templating engine
- Spring Boot Dev Tools: fast reload/restart, and enhanced configurations.
Then, click on Finish
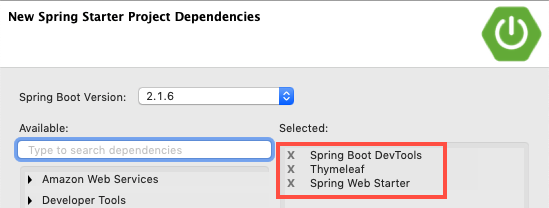
Look in the source folder, where you can find the generated Application
, annotated as @SpringBootApplication
.
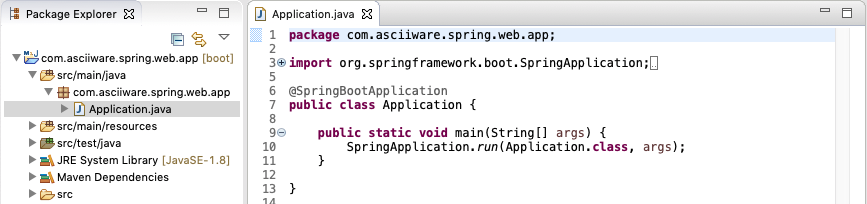
You can now run the Spring application, which runs at http://localhost:8080/. As the application is empty, you won’t see any result when you open the page in a browser.
Running a Spring Boot Web App
To run the SpringBoot Application select the Application
class, right-click on it, and select Run As.. > Java Application.
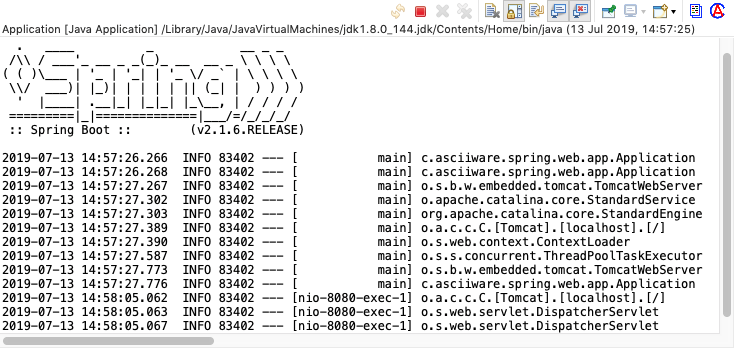
After launching main()
, we see in the console a tomcat server is started on port 8080. Here is what happened:
main()
callsSpringApplication.run(..)
that starts Spring, instantiate the Context and set up controllers and other components.- The
Application
, annotated as@SpringBootApplication
, is wrapped with a default configuration - Spring computes the configuration and starts an embedded tomcat to serve the application’s content.
Add a controller
In this minimal example, the backend is made of a single controller receiving GET requests on http://localhost:8080/test and returning a simple String as a response.
A Controller is a Spring component to handle web requests received by the application.
To add a controller to the Spring Application, we add a class AppTestController
and annotate the class as @Controller
.
@Controller public class AppTestController { @GetMapping("/test") @ResponseBody public String testMessage(@RequestParam(name="msg", required=false, defaultValue = "NONE") String message) { return "message received from the backend: " + message; } }
@GetMapping("/test")
: ensures the GET requests to “/test’ are mapped to the testMessage method.@RequestParam
binds the value of query string parameters to actual variables on the method, providing also a default value to it.@ResponseBody
indicates the return type can be written directly into the http response
Now, if you open the address http://localhost:8080/test?msg=Hello, World! in your browser, you can see a trivial backend response.

Real applications don’t serve backend data directly to the user. Indeed, we added @ResponseBody
just to see some output from the web application.
Now remove the @ResponseBody
annotation from testMessage()
and reload the page in your browser. You will see an error message
Add Presentation (to be continued..)
A real web app does not serve the backend content directly, but keeps the information into a model and typically serves content using a frontend.
[insert-updated-content]
If you reload the page now, you’ll notice an error both in the webpage and in the console. That’s because, by default, Spring is searching
What happens when we run our SpringBoot application? Spring has a lot of components, and SpringBoot is a convention over configuration approach to run an application with default modules loaded.
References
- Serving Web Content with Spring MVC, Spring Website
0 Comments