JSP and JSTL
The JSP Standard Taglibrary (JSTL) is a set of libraries providing dynamic tags helping to perform common JSP operations, such as store/display data, perform iterations, manupulate XML, perform I/O, etc.
In this post we see a few simple examples on how to use JSTL core taglibs.
JSTL
JSTL (JSP Standard Tag Library) is a specification for Java EE Web application development ( JCP spec. JSR-52). JSTL extends the JSP specification by adding a tag library of JSP tags for common tasks, such as XML data processing, conditional execution, loops, etc.
JSTL provides an effective way to embed logic within a JSP page without using Java code directly. This lead to develop code that is more maintainable code and enables separation of concerns between application code and user interface.
Install JSTL
To use JSTL, you need both to install TLDs (TagLibrary Descriptors) and the implementation of such libraries.
In this case, we’re going to install JSTL by adding to the classpath a single library containing both TLDs and the tag’s implementations.

You can find jstl-1.2.jar on the web or from this local archive.
Test JSTL
The provided jar contains both the libraries and the TLD definitions. So, we just need to put the library in the classpath and we’re free to use the JSTL.
To use the JSTL, first, we need to declare the usage of the taglibs on top of the JSP page, associating the taglib to a specific namespace prefix, in this case c:
.
<!-- declare usage of common -->
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
Then we could use the JSTL tags in the page, as per example below.
<h1>Test JSTL</h1>
<!-- import an xml file in a variable, then prints out to page -->
<c:import url="test.xml" var="xml"/>
1. <c:out value="${xml}"/><br/>
2. <c:out value="${xml}" escapeXml="false"/><br/>
In the snippet above we used:
- c:import to read a file on the server, and store its content inside a variable
- c:out to output the content of a variable to the page (in the example, we escape xml)
Run the server, and you should see a result like the image below.
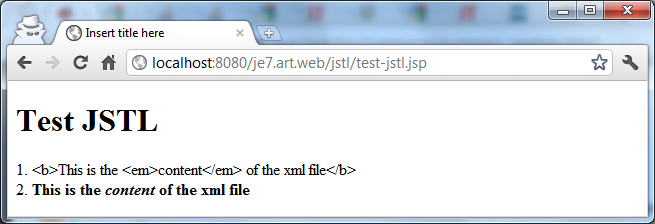
Below, you see some further tests for the jstl /core libraries.
In the first snippet, we demonstrate the usage of a for loop to build a table.
<table border="1">
<tr>
<th>i:</th>
<c:forEach var="i" begin="1" end="10" step="1">
<td><c:out value="${i}"/></td>
</c:forEach>
</tr>
</table>
In the second, we list all the parameters available for the JSP. Note: here the parameters are provided with the implicit parameter object, that is an instance of map.
<ul>
<c:forEach var="pageParameters" items="${param}">
<li><c:out value="${pageParameters.key}"/> =
<c:out value="${pageParameters.value}"/></li>
</c:forEach>
</ul>
See the results in the image below.
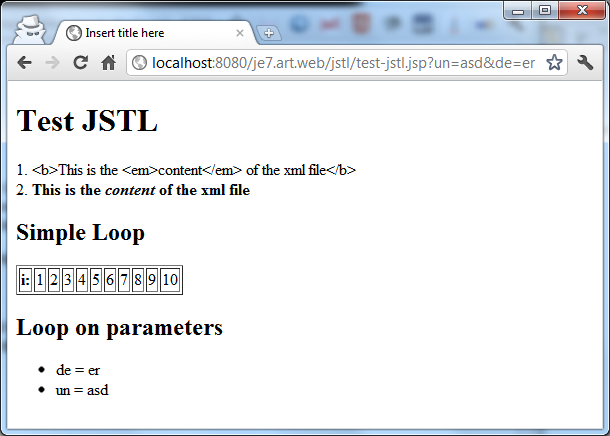
References
0 Comments