This article aims to provide a comprehensive understanding of API Gateways, their benefits, and practical implementation strategies, with a focus on Java-based solutions.
Introduction
An API Gateway is an abstraction layer representing a single entry-point for a system providing multiple services.
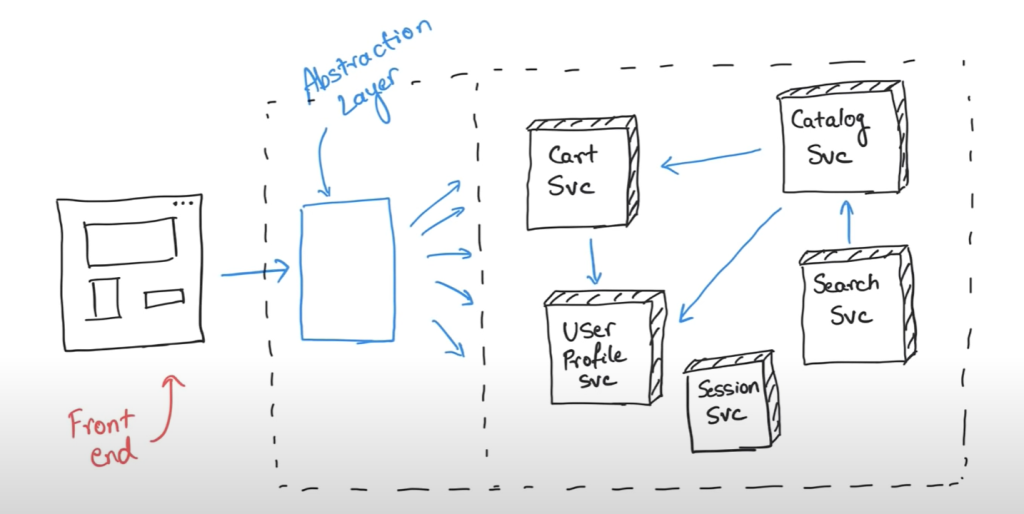
In the world of microservices and distributed systems, API Gateways have become an essential component for managing and optimizing communications between client and backend services.
What is an API Gateway?
An API Gateway is an architectural pattern that serves as a single entry point for a collection of microservices. It acts as an intermediary layer between clients (such as web or mobile applications) and the backend services, providing a unified interface for accessing various functionalities.
Key features of an API Gateway include:
- Single entry point for multiple services
- Request routing and aggregation
- Protocol translation
- Authentication and security
- Monitoring and analytics
- Rate limiting and throttling
Benefits of using an API Gateway
- Simplify client side development: Clients interact with a single endpoint, reducing complexity in frontend development.
- Encapsulate internals: The gateway hides the details of the microservices architecture from external clients.
- Enhanche security: Centralized anthentication and authorization can be implemented at the gateway level.
- Improve Performances: By aggregating multiple calls, an API Gateway can reduce the number of round trips between the client and the backend.
- Easy monitoring and analytics: A single entry point allows for centralized logging, monitoring, and analytics of all API traffic.
- Flexible scaling: Different components can be scaled independently based on their specific requirements.
Implementing an API Gateway
We focus on a Java-based solution, specifically using Spring Cloud Gateway, which is a popular choice for Java Developers.
Spring Cloud Gateway
Spring Cloud Gateway is a powerful solution built on top of Spring Boot, Spring WebFlux, and Project Reactor, offering a reactive and non-blocking approach to handling requests.
Here is a basic example of how to set up a Spring Cloud Gateway:
@SpringBootApplication
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("service1_route", r -> r.path("/service1/**")
.uri("http://localhost:8081"))
.route("service2_route", r -> r.path("/service2/**")
.uri("http://localhost:8082"))
.build();
}
}
In thie example, we’re creating two routes:
- Requests to
/service1/**
are routed tohttp://localhost:8081
- Requests to
/service2/**
are routed tohttp://localhost:8082
Adding Authentication
Below is an example configuration to set op OAuth2 authentication for the API Gateway
@Bean
public SecurityWebFilterChain springSecurityFilterChain(ServerHttpSecurity http) {
return http.csrf().disable()
.authorizeExchange()
.pathMatchers("/public/**").permitAll()
.anyExchange().authenticated()
.and()
.oauth2Login()
.and()
.oauth2ResourceServer()
.jwt().and().and().build();
}
Implementing Rate Limiting
Below is an example to add rate limiting to the /api/**
route, allowing one request per second with a burst capacity of two.
@Bean
KeyResolver userKeyResolver() {
return exchange -> Mono.just(exchange.getRequest().getRemoteAddress().getAddress().getHostAddress());
}
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("rate_limited_route", r -> r.path("/api/**")
.filters(f -> f.requestRateLimiter(c -> c.setRateLimiter(redisRateLimiter())))
.uri("http://localhost:8081"))
.build();
}
@Bean
public RedisRateLimiter redisRateLimiter() {
return new RedisRateLimiter(1, 2);
}
Best Practices for API Gateway Implementation
Here is a list of best practices to consider
- Design for failure: Implement circuit breakers and fallback mechanisms to handle service failures gracefully
- Caching: use caching strategies to improve performance and reduce load on backend services
- Versioning: Implement API versioning to manage changes and updates without breaking existing clients.
- Monitoring and Logging: Set up comprehensive logging and monitoring to track performance and identity issue quickly.
- Security: Implement robust security measures, including authentication, authorization, and encryption.
- Performance Optimization: Continuosly monitor and optimize the gateway’s performance to prevent it from becoming a bottleneck.
Conclusion
API Gateways are a crucial component in modern, distributed architectures. They provide a single entry point for clients, simplifying frontend development while offering powerful tools for managing, secusing, and optimizing backend services. By leveraging solutions like Spring Cloud Gateway, Java developers can efficiently implement and manage API Gateways, enabling more scalable and maintainable microservices architectures.
Remember: API Gateway offer a number of benefit, but they also introduce additional complexity and potential points of failures. Careful planning, implementation, and continuous management are essential to take full advantage of this architectural pattern.
0 Comments