This post details the architecture of the Routine App, starting from the goals, and diving into the tech stack, development stages and strategies to ensure the project can be implemented with high-quality standards.
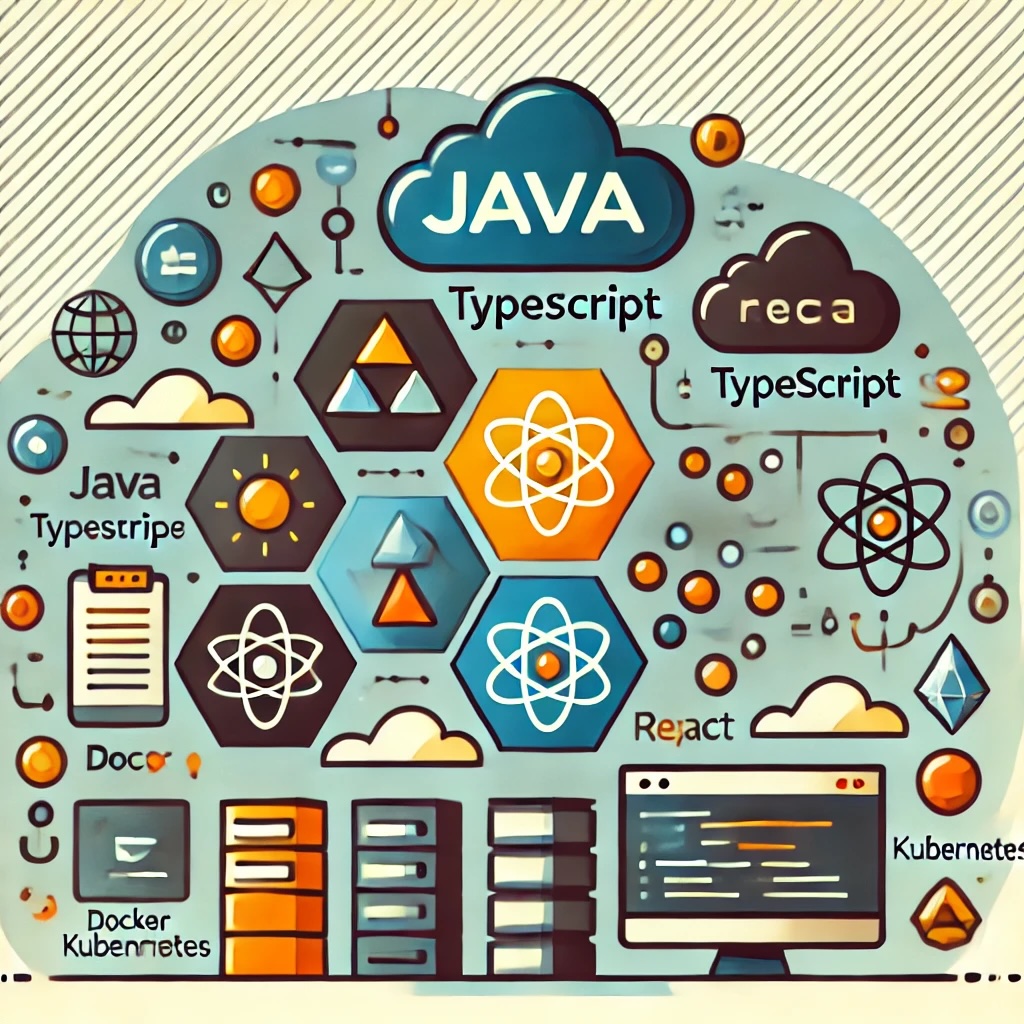
Project Overview
Goals
- Showcase abilities in Java, Cloud, and TypeScript.
- Build a minimal app with low code, designed to be easy to maintain, document, and reuse as a template for future applications.
- Release a free MVP and monetize through premium features.
Technology Stack
- Backend: Java Microservices (Spring Boot).
- Frontend Web: React (TypeScript).
- Frontend Mobile: React Native (TypeScript).
Development Approach
- Solo development, using AI for assistance in coding, testing, and documentation.
- Focus on incremental feature implementation with CI/CD pipelines using GitHub Actions.
System Architecture
1. Backend Architecture
- Spring Boot Microservices:
- Spring Boot is selected due to its community support, stability, and ease of use for microservices architecture.
- Microservices will be connected via REST or GraphQL for efficient data querying.
- Event-Driven Messaging:
- The system will use RabbitMQ for messaging, providing asynchronous communication between services.
- Why RabbitMQ?: Simpler to set up and operate than Apache Kafka, which is more suited for high-throughput, fault-tolerant systems.
- Database Setup:
- PostgreSQL for relational data (tasks, calendar events).
- MongoDB may be added later for flexible data storage (user preferences).
- Initial setup will only use PostgreSQL to avoid complexity.
- Authentication:
- OAuth 2.0 via Spring Security will be used for authentication, especially to integrate with Google Calendar for syncing.
2. Frontend Architecture
- Web Frontend:
- Built using React with TypeScript for a modern, scalable single-page application (SPA).
- No need for Next.js as there’s no immediate need for server-side rendering (SSR).
- Mobile Frontend:
- React Native for mobile development, allowing the reuse of TypeScript code from the web app.
- The mobile app will be built after the web version reaches a stable state, ensuring core features are working.
3. API Gateway & Communication
- API Gateway will be used to:
- Handle routing of requests to different microservices.
- Provide authentication, rate-limiting, and logging.
- Manage cross-cutting concerns like monitoring and security.
4. Message Queue
- RabbitMQ will be the messaging queue for handling:
- Event-driven communication between services (e.g., task creation, calendar event updates).
- Asynchronous task scheduling, allowing services to remain decoupled and scalable.
5. Containerization & Orchestration
- Docker will be used for containerizing each microservice.
- Kubernetes will handle orchestration, providing automated scaling, management, and load balancing.
- Note: Kubernetes will be implemented later in the project when scaling becomes necessary.
Development Stages
Stage 0: Initial Prototype (Monolithic)
- Backend:
- Spring Boot monolithic application
- RESTful API endpoints
- Single PostgreSQL database
- Basic Spring Security for authentication
- Frontend:
- React Single Page Application (SPA)
- Basic state management (e.g., React Context or Redux)
- Mobile:
- Not implemented in this stage
- CI/CD:
- GitHub Actions workflow for building and testing
- Manual deployment to a cloud platform (e.g., Heroku)
Stage 1: MVP (Modular Monolith)
- Backend
- Refactor Monolith into modules as needed
- Develop the Task Service and Calendar Service initially, focusing on functionality like managing tasks and syncing with Google Calendar.
- Web Frontend:
- Complete the React-based web frontend, and test the use cases. This will interface with the backend services to provide task management and calendar functionalities.
- CI/CD with GitHub Actions:
- GitHub Actions will handle continuous integration, testing, and deployment.
- Different automated pipelines to test code on commits, run unit tests, and deploy the application to staging environments.
Stage 2: Scaling to Microservices
- Refactor Backend to Microservices:
- Once the core features (task management, calendar sync) are stable, split the monolithic backend into microservices for:
- Task Service
- Calendar Service
- Sync Service (for Google Calendar integration)
- User Service (for authentication)
- Mobile Frontend:
- After the web frontend stabilizes, develop the mobile app using React Native.
- Ensure that both web and mobile frontends share a common codebase where possible to avoid duplicating effort.
- Containerization:
- Start using Docker early to containerize each microservice, ensuring consistency across development and production environments.
- Expand the CI/CD pipeline to deploy containers to a Docker-based staging environment.
Stage 3: Scalability and Commercial Expansion
- Scalability:
- Implement Kubernetes for orchestrating containers, ensuring the application can scale with increased traffic.
- Add Kafka if messaging requirements grow and RabbitMQ becomes a bottleneck, though this is optional based on user demand and traffic.
- Security:
- Implement advanced security features like JWT tokens, OAuth scopes, and API rate limiting as user base increases.
- Premium Features:
- Once the MVP is stable and launched, premium features (advanced calendar sync, NLP, etc.) will be incrementally added.
Critical Considerations
Cross-Platform Development
- Ensure that the mobile and web apps are developed and tested in parallel, especially as both rely on shared backend services.
- Keep the user interface consistent across platforms to provide a seamless experience.
Code Quality
- While AI will assist in code generation, it is essential to manually review critical parts of the code to maintain high quality.
- Use SonarQube or similar tools integrated with the CI pipeline for continuous code quality checks.
Documentation & Demo
- As a solo developer, make sure to maintain up-to-date documentation in the form of GitHub README files, blog posts, and demo videos.
- Regularly update a demo app deployed to a public environment (e.g., Heroku or AWS) for showcasing your progress to potential users or clients.
Summary of Development Flow
- Start with a Monolithic Backend: Build a single backend application with key features like task management and calendar sync.
- Develop React Web Frontend: Connect the frontend to the backend via REST/GraphQL APIs.
- Set up GitHub Actions: Use automated pipelines for continuous integration, deployment, and testing.
- Refactor to Microservices: First move to a modular monolith, and then split the backend into individual services once core features are stable.
- Introduce Mobile App: Build the React Native mobile app after the web frontend is fully functional.
- Implement Docker & Kubernetes: Containerize services and use Kubernetes for orchestration in the later stages.
- Deploy and Scale: Focus on performance, scalability, and premium features post-MVP launch.
0 Comments