The Routine App is an ambitious project aimed at creating a productivity tool while showcasing advanced software development skills. This article outlines the primary goals, and key features of the Routine App, setting the foundation for the entire development process.
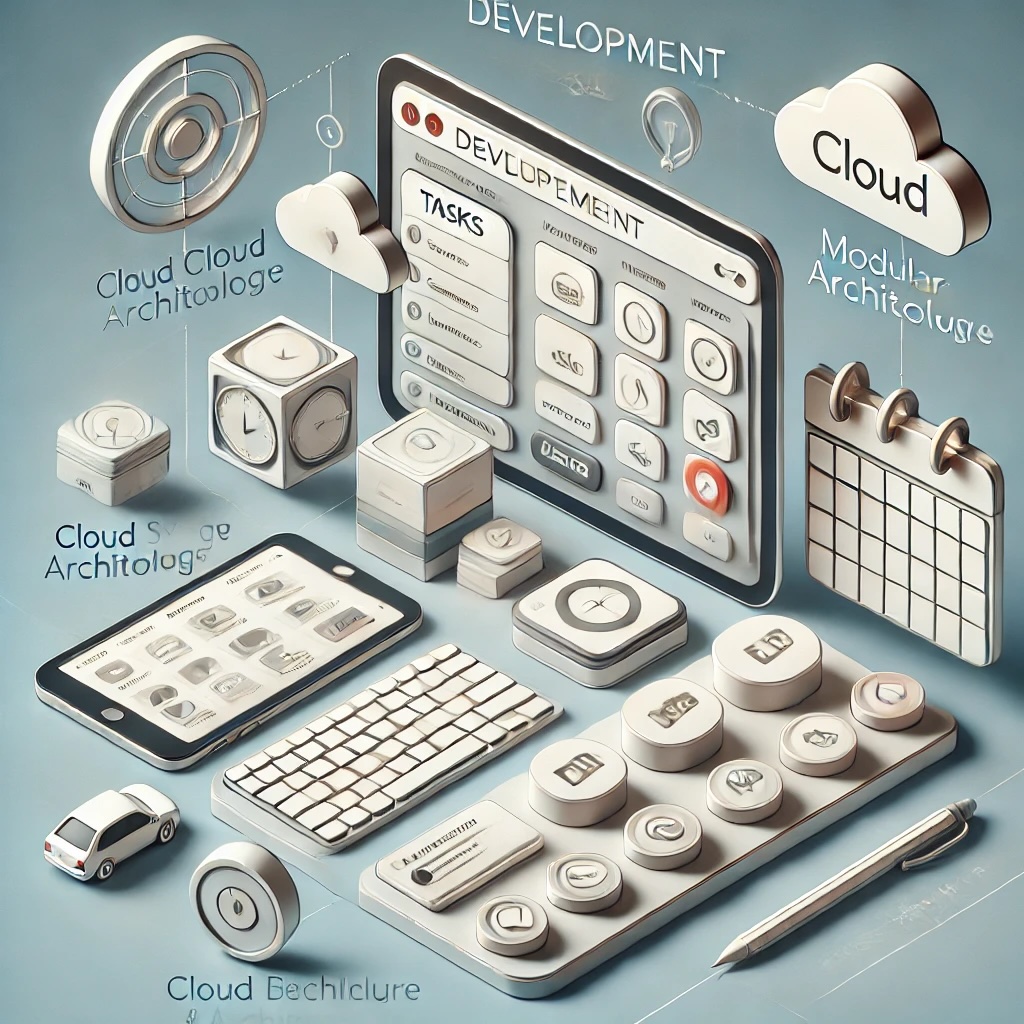
This case study will take you through the entire process of creating the Routine App, from initial concept to a fully-scaled application. Each development stage is covered by a different post, which will highlight key decisions, challenges, and solutions along the way:
- Routine App Case Study: (this page) to present the use cases, consider the constraints, and define the project parameters.
- Routine App Architecture
- Routine App Prototype Backend
- Routine App Prototypr Frontend
- Routine App MVP
- Routine App Scaling
- Routine App Deployment
The remainder of this document explains the use case, starting from its inception to the collection of requirements
Primary Objectives
Goal: create a productivity tool accessible via web/mobile. The MVP should be distributed for free, while value-added feature should be sold to the public. Given my full-stack expertise, I am bound to use a stack including Java, Typescript, and Microservice + Cloud technologies.
Implementation: Minimal and low-code, aiming to be high-quality and easy to maintain. The implementation should be modular and well documented in order to maximize module reusability in future projects, and process reproducibility for the readers.
Initial Idea
Let’s start with a concrete idea of the stack to implement, in order to come up with possible alternatives.
- Backend
- Java-based microservices using Spring Boot or Quarkus, connected via REST or GraphQL
- Use event-driven communication between services for asynchronous task scheduling (Apache Kafka or RabbitMQ)
- DataBase:
- relational database (e.g. PostgreSQL) for structured data (e.g. calendar events, tasks)
- noSQL database (e.g. MongoDB) for more flexible data storage like user preferences
- Authentication: OAuth 2.0 Integration for Google Calendar Sync (Spring Security OAuth)
- Frontend
- Typescript using React or Next.js for component-based architecture. These frameworks are highly scalable and have strong community support.
- Frontend Mobile
- Considering react Native (which allows to share much of the Typescript codebase) or Flutter (for faster performances and native-like apps) for both Android and iOS apps. These technologies allows to create cross-platform apps with one codebase which is efficient
- React Native (for reuse much of Typescript Codebase) or Flutter (for fast and native-like apps).
Architecture
The initial architectural decisions are tailored to achieve the set goals in the specific context.
- Backend: SpringBoot or Quarkus? SpringBoot is more widely used, has excellent docs and large community
- Event-driven Messaging: Kafka or RabbitMQ? RabbitMQ, as it is simpler to set up and operate, despite Kafka is ideal for high-throughput, dist-systems with need for scalability and fault tolerance.
- Frontend React vs Next.js: Plain React is simpler to start, and provide all the features needed.
- Mobile React Native vs Flutter: React Native, since React is used in b/e, this will allow to share more code, speeding up development
- Implementation strategy: Start small, implement gradually, use CI/CD, use AI for code, testing, docs
The main criticality is the complexity for a solo developer, which can be addressed as follows:
- Start with a monolithic backend and gradually modularize it into microservices as needed -> fast MVP, avoiding overhead of managing multiple services
- Initially use a single DB (PostgreSQL) and only add MongoDB when necessary
- Delay implementing Kubernetes until the app requires that level of orchestration
- Cross-platform issues: While React Native allows sharing a codebase, mobile-specific features might need careful handling. Test the web and mobile frontends in parallel to avoid integration issues later.
Project Management
A lean management style is the most appropriate for this solo project. An agile board for task tracking with short sprints with 2 weeks iterations should be good for starting. It will be important to perform regular self-reviews to assess progress and adjust priorities.
- Board accessible on GitHub: psuzzi’s Routine
Development time is limited, and based on scope, feature complexity and experience, a ballpark estimate would be:
- Initial setup: 2-4 weeks, including Setting up Spring Boot backend, React Frontend and CI/CD pipeline
- MVP: 3-4 months of focused development. This is considering solo development and using AI-assist for code generation, testing and documentation.
- Premium features and fine-tuning would likely add additional 2-3 months.
Here is a list of ideas for having better estimate and time tracking, and to measure progress:
- Break down features into small, estimable tasks that can be timeboxed in 2-weeks sprints
- Use story points for estimation, and calculate avg. velocity in SP/sprint
- Set sprint milestones at the end of each sprint to measure the progress towards MVP completion
- Track time spent on each task (dog-fooding?: use the time tracking sw for its own TT)
- Reassess progress at the end of each Sprint by using borndown charts to visualize progress
- Readjust roadmap to ensure critical features stay on track
Implementation
Initial prototyping strategy
Focus on setting up the core infrastructure. The aim is to create a working prototype.
- Start with a monolithic web frontend, then extend to mobile once core features are stable
- Implement features incrementally, starting with the most critical ones
- Use CI/CD from the beginning to streamline the deployment process
- Leverage AI for code generation, testing, and documentation to increase velocity
To maintain high-velocity:
Mid-development strategy
When the project is underway, focus on the MVP while refining the codebase.
- Start with core backend services: Task service and calendar service, then move to sync service for google calendar integration. These are the core components, which need testing in isolation
- Develop the frontend SPA simultaneously: Once the core backend services are up, set up the web frontend to interface with the backend APIs. This gives a feedback loop for quick testing and iteration.
- Implement the mobile frontend later: After the web frontend is stable, shift to mobile development using React Native
- Leverage containerization early: use docker to containerize each microservice early in the development process. It will help to easily test and deploy services in parallel
- Prioritize a working MVP: Keep MVP features in focus and avoid spending time on additional features until the core app is stable.
Commercial Expansion Strategy
As the application becomes commercial and more members are involved, development shifts toward scalability, security, and maintainability. The project is modularized to allow parallel development. Infrastructure moves towards cloud-based solutions with microservices and containerization to handle increasing loads, while proper documentation , team collaboration tools, and code review processes are enforced to maintain quality.
Criticalities
- Ensure the monolithic backend is designed with future microservices in mind to ease transition.
- Start with a minimal viable CI/CD pipeline and expand as needed
- Consider implementing basic containerization early, even before microservices, to familiarize the project with the tech.
- Over-reliance on AI: While AI tools can assist with code, testing and documentation, ensure manual reviews and validation of critical parts of the code to maintain quality and avoid bugs in production.
- Cross-platform pitfalls: Ensure continuous integration and testing for both web and mobile frontends to avoid misaligned user experiences across platforms
Business
Tiered Features, Ordered and estimated
Plan: reach market early with a free MVP. Add incrementally new features, until we can ask payment for premium ones.
Features: divided in five tiers, from most important for the MPV (Tier-1) to least important user features (Tier-5)
Tiers: in each tier the features should be ordered from most to least important.
Estimation: Complexity with Fibonacci numbers. E.g. 1, 2, 3, 5, 8 -> Very Low, Low, Medium, High, Very High.
Note: implementation of some features will be documented as blog posts and videos, with the aim to showcase a portfolio, and sell additional services.
Note: the below features are added to GitHub: psuzzi’s Routine board.
Tier-1: MVP Features
Must-have features for an MVP to get the app functional and market-ready
- (1) Todo list functionality: core task management
- (3, maybe5?) Basic Calendar integration: sync tasks and events with Google Calendar
- (5) Concise language for task/event declarations: Simple syntax for input (killer feature if it’s intuitive).
- (5, maybe8?) Cross-platform support: Large audience web||mobile -> ensure seamless usage across platforms.
- (3>2) User Authentication and Authorization: secure user accounts and authentication
- (3) Basic notifications and reminders: alerts, email, non-configurable.
Tier-2: Strategic features
Strategic features, critical to transform the MVP into a real application with Premium features.
- (3) Data Sync across devices: Ensure seamless experience across web and mobile platforms
- (2) Search Functionality: to quickly find tasks and events
- (3, maybe5?) Offline Support: Local caching and syncing for offline use (in par with many productivity apps)
Tier-3: Premium, Value-add
Enhance user experience and provide value the users are willing to pay for (premium features)
- (5) Advanced calendar sync: including external calendars and Google Calendar
- (3) Notifications and Reminders improvement: user expect timely reminder for tasks and calendar events, preferably with custom settings. (configuration language?)
- (2, maybe 3?) Customizable Views and Themes: Different calendar/task views (day, week, month / priority-based, due-date based) and visual customization.
- (3, maybe 5?) Task Dependencies and Prioritization: manage task hierarchies and priorities (e.g. Task B start when Task A is complete) could be a powerful feature.
- (5, maybe 8) NLP (Natural Language Processing): Enhance the concise language feature by implementgin NLP to interpret user input more intelligently
Tier-4 Nice-to-have
Other relevant features, that can be nice to have
- (?) Customizable notifications: allow users to set reminders for tasks and events
- (2) Recurring Tasks and events: support for creating repeating todo items and calendar events
- (2) Tags and Categories: allow user to organize tasks and events with custom tags or predefined categories
- (?) Priority Levels: Implement a system for user to prioritize tasks
- (3, maybe 5) Data Visualization: chart/graphs for productivity insights. E.g. task completion rates, busy times, etc.
- (5) Collaboration Features: share tasks/calendars with others (users, team, family)
Tier-5 Future Enhancements
Tier to collect user features and other features to be considered for development
- (?) Time tracking: features comparable to clockify
- (?) Graphical editing on the task list
0 Comments